Bluetooth functionality for the Smart Aquarium is done between an Android app and the Arduino Nano 33 BLE, meaning we need some Arduino code for sending information as well as a good amount of Android code to connect and receive this information.
The arduino code is almost complete (shown below), though the sensors inputs will need to be correctly calculated and possibly adjusted for.
Sending the sensor information through plaintext should not be an issue, so the arduino will do the calculation and send the numbers straight to the Android device.
const int mVolt = 3300; const int tmpPin = A0; const int phPin = A1; const int waterPin = A2; float tmpVal, phVal, waterVal = 0; void setup() { Serial.begin(9600); } void loop() { int vin = 0; //read input voltage from analog pin and calculate the value based on formula vin = analogRead(tmpPin); tmpVal= (vin*(mVolt/1024)-500)/10; vin = analogRead(phPin); phVal = vin; vin = analogRead(waterPin); waterVal = vin; //write results to serial port (Nano 33 BLE serial is bluetooth) Serial.print("Example temperature reading: "); Serial.println(tmpVal, 5); Serial.print("Example PH reading: "); Serial.println(phVal, 2); Serial.print("Example flood reading: "); Serial.println(waterVal); }
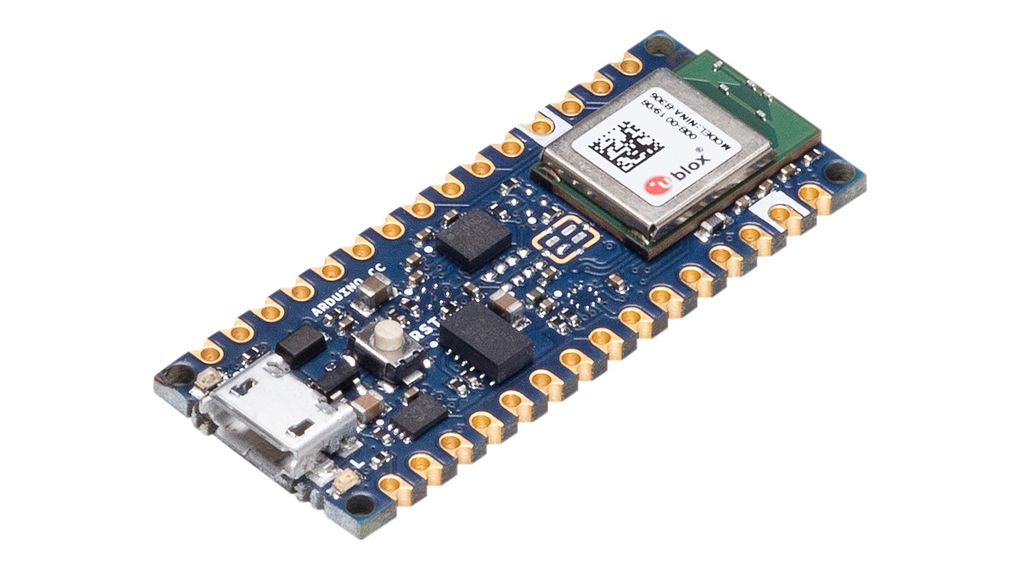
On the receiving end, Bluetooth Low Energy code appears to be a bit complicated compared to normal Bluetooth, as BLE works a bit more like a messageboard that a device can read rather than a typical connection.
At the moment, Kasper is working on the Android code to make the Bluetooth stuff all work, the code below is a rough idea and is definitely not functional yet. All the code really needs to do is read the information broadcasted by the Nano.
Learning how to code BLE/Android in between everything going on as well as other projects means we are a bit behind on this part.
private val bluetoothLeScanner = BluetoothAdapter.getDefaultAdapter().bluetoothLeScanner private var mScanning = false private val handler = Handler() private val SCAN_PERIOD: Long = 10000 fun connectBLE() { val bluetoothManager = getSystemService(Context.BLUETOOTH_SERVICE) as BluetoothManager val bluetoothAdapter = bluetoothManager.adapter if (bluetoothAdapter == null || !bluetoothAdapter.isEnabled) { val enableBtIntent = Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE) startActivityForResult(enableBtIntent, REQUEST_ENABLE_BT) } } private fun scanLeDevice() { if (!mScanning) { handler.postDelayed({ mScanning = false bluetoothLeScanner.stopScan(leScanCallback) }, SCAN_PERIOD) mScanning = true bluetoothLeScanner.startScan(leScanCallback) } else { mScanning = false bluetoothLeScanner.stopScan(leScanCallback) } } fun close() { bluetoothGatt?.close() bluetoothGatt = null }